Optimizing React Performance with Lazy Loading and Code Splitting
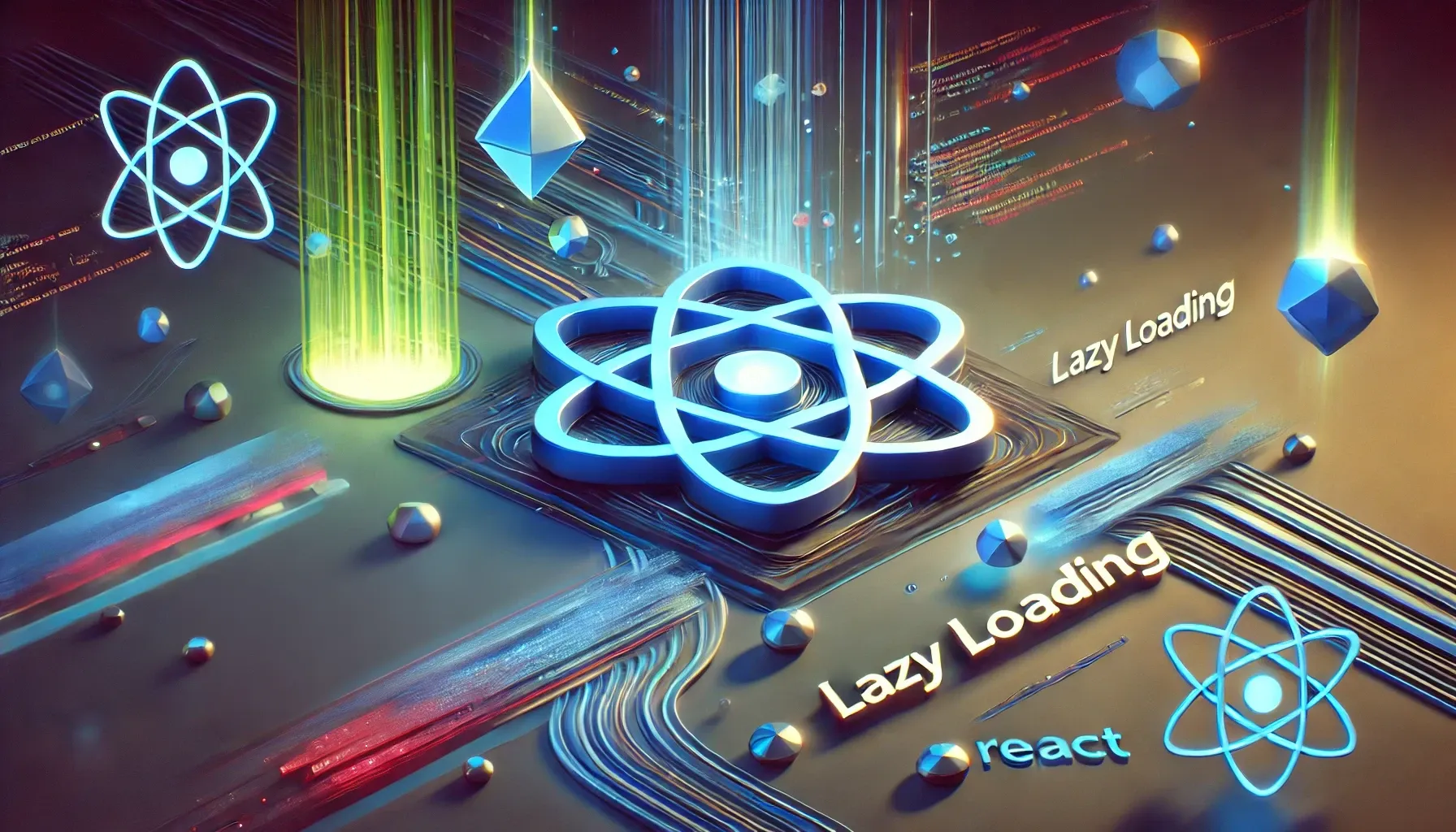
Optimizing React Performance with Lazy Loading and Code Splitting
In React, performance is crucial, especially for larger apps. Lazy loading and code splitting are two powerful techniques that help improve your app's speed by loading only what the user needs when they need it. Here's how you can optimize your React app with these techniques.
1. Lazy Loading Pages (Routes) with React.lazy()
and Suspense
In React applications, routing plays a significant role in determining how users navigate between different views or pages. Loading all the routes upfront can lead to larger bundles and slower initial load times. This is where lazy loading pages using React.lazy()
and Suspense
comes in.
With lazy loading, you can dynamically load each page only when a user navigates to it. This approach defers loading non-essential code until it's absolutely necessary, improving your app's First Contentful Paint (FCP) and overall performance.
Example:
import { BrowserRouter as Router, Route, Routes } from 'react-router-dom';
import { Suspense, lazy } from 'react';
const Home = lazy(() => import('./pages/Home'));
const About = lazy(() => import('./pages/About'));
const Contact = lazy(() => import('./pages/Contact'));
const App = () => {
return (
<Router>
<Suspense fallback={<div>Loading page...</div>}>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
<Route path="/contact" element={<Contact />} />
</Routes>
</Suspense>
</Router>
);
};
export default App;
How It Works:
React.lazy()
: Dynamically imports a module (in this case, a page component) when it's needed, enabling code splitting.Suspense
: Wraps the lazy-loaded component and provides a fallback UI (such as a loading spinner) while the component is being fetched.
This approach ensures that pages are not bundled into the initial JavaScript file, significantly reducing the bundle size and load time for the first page. As users navigate, the app fetches the additional pages, loading them in the background.
2. Lazy Loading In-Page Components
In addition to lazy loading routes, you can apply the same technique to in-page components. If certain components, such as heavy charts, third-party libraries, or large UI elements, are not essential for the initial view, you can lazy load them to further improve performance.
Lazy loading in-page components works similarly to routes, and it's another excellent way to perform code splitting. This ensures that only critical components are loaded upfront, while non-critical ones load as needed.
Example:
import React, { Suspense, lazy } from 'react';
const HeavyComponent = lazy(() => import('./components/HeavyComponent'));
const Home = () => {
return (
<div>
<h1>Welcome to the Homepage</h1>
{/* Lazy load HeavyComponent */}
<Suspense fallback={<div>Loading component...</div>}>
<HeavyComponent />
</Suspense>
</div>
);
};
export default Home;
Why This Matters:
- Code Splitting: By using
React.lazy()
for individual components, you create multiple smaller bundles, improving both the initial load time and the user experience. - Fallback UI:
Suspense
provides a mechanism to show a fallback while the component is being loaded, ensuring that the user isn’t left with a blank screen.
Other Ways to Optimize Performance
Beyond lazy loading and code splitting for routes and components, there are several other strategies to enhance the performance of your React application:
3. Lazy Loading Images and Media
Large images can delay page load times, especially if they are above the fold or critical for the user experience. By using the loading="lazy"
attribute, images will only load when they are about to enter the viewport, improving page speed and reducing the initial download size.
<img src={exampleImage} alt="Example" loading="lazy" />
- For background images, consider Low-Quality Image Placeholders (LQIP), where a low-resolution image is shown first, followed by the full image after it loads.
- Preloading critical images can also help ensure key visuals are ready faster without blocking rendering.
4. Lazy Loading Iframes and Videos
For media-heavy elements like iframes and videos, you can use the IntersectionObserver API to load these elements only when they are visible in the viewport. This prevents heavy content from blocking the page rendering process.
import { useState, useEffect, useRef } from 'react';
const LazyIframe = () => {
const [isLoaded, setIsLoaded] = useState(false);
const iframeRef = useRef(null);
useEffect(() => {
const observer = new IntersectionObserver((entries) => {
entries.forEach((entry) => {
if (entry.isIntersecting) {
setIsLoaded(true);
observer.disconnect();
}
});
});
if (iframeRef.current) {
observer.observe(iframeRef.current);
}
return () => {
if (iframeRef.current) {
observer.unobserve(iframeRef.current);
}
};
}, []);
return (
<div ref={iframeRef}>
{isLoaded ? (
<iframe
src="https://www.example.com"
width="600"
height="400"
title="Lazy Loaded Iframe"
></iframe>
) : (
<div>Loading iframe...</div>
)}
</div>
);
};
export default LazyIframe;
5. Compress and Optimize Images
While lazy loading images is essential, reducing their size with compression tools like TinyPNG or Squoosh can dramatically reduce their download size without affecting quality. WebP is a preferred image format for web performance due to its superior compression compared to traditional formats like JPEG or PNG.
6. Defer Non-Essential JavaScript and CSS
To further optimize performance, you can defer non-essential JavaScript and split CSS. Ensure that only critical CSS is loaded in the initial render and defer non-critical styles or JavaScript until after the page has loaded. This technique is particularly useful for scripts that don't affect the initial rendering of the page.
Conclusion
By implementing lazy loading and code splitting with React.lazy()
and Suspense
, you can significantly enhance the performance of your React applications. These techniques reduce the initial load time by breaking the app into smaller chunks and loading only the necessary components and routes when needed.
Additionally, lazy loading images, media, and optimizing assets like JavaScript and CSS will further improve the user experience, making your site faster and more responsive.
Take advantage of these strategies to deliver a performant and efficient web experience!